Cropper
Preview and crop image
Usage
Simple Usage
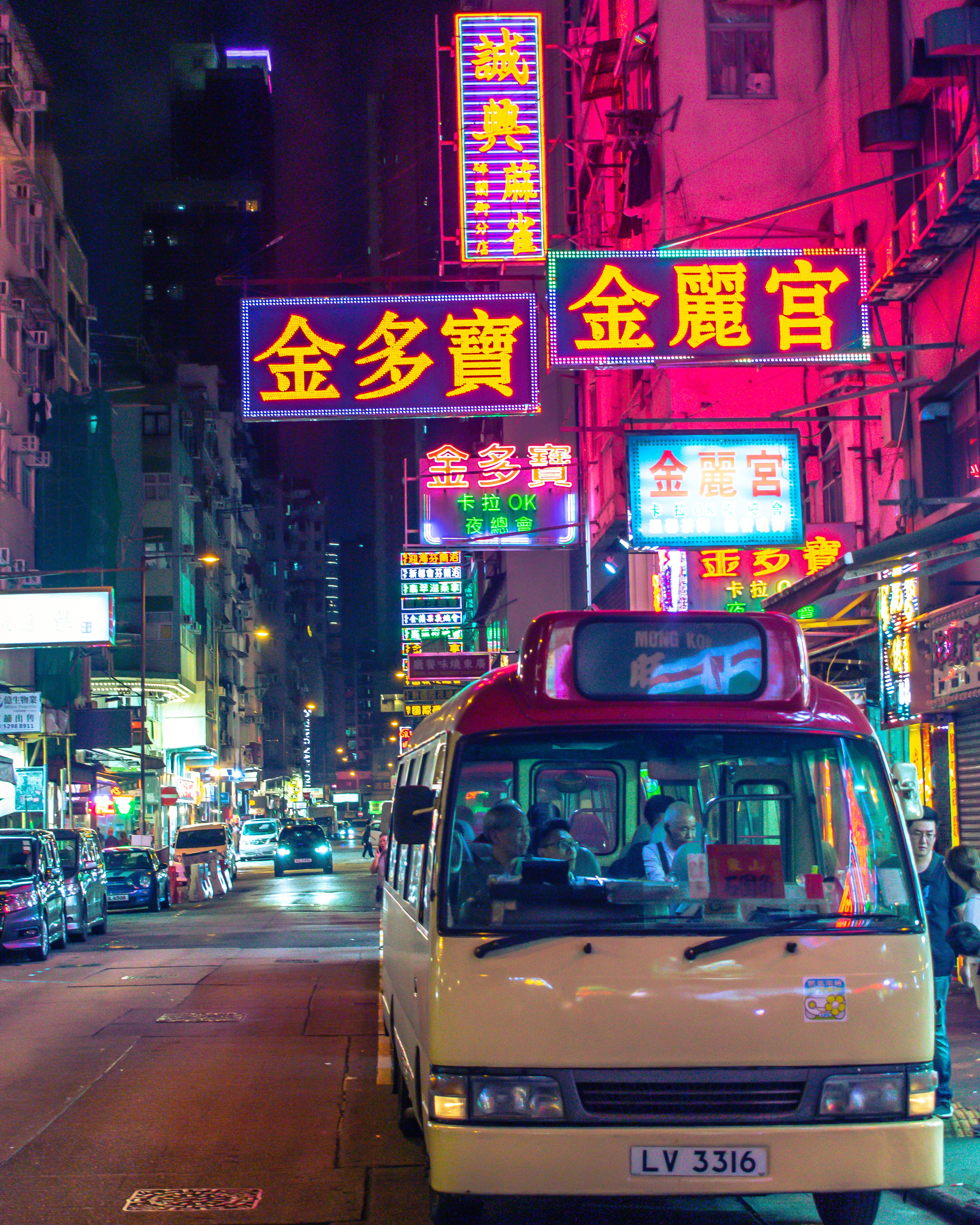
vue
<template>
<p-cropper src="./assets/sample-1.jpg" />
</template>
Crop Size
You can adjust crop size using width
, height
or ratio
.
Using width
and height
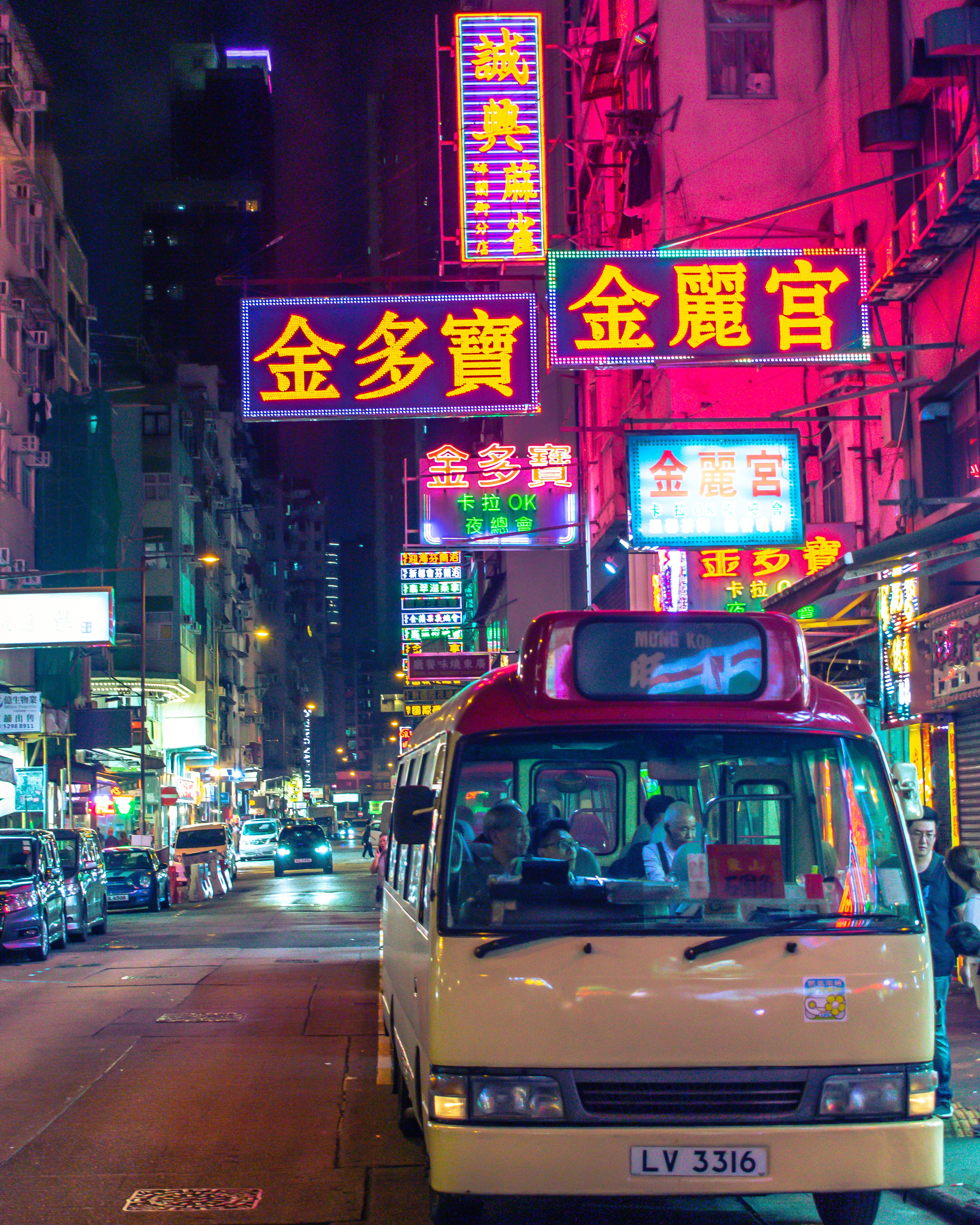
vue
<template>
<p-cropper src="./assets/sample-1.jpg" width="500" height="200" />
</template>
Using Ratio
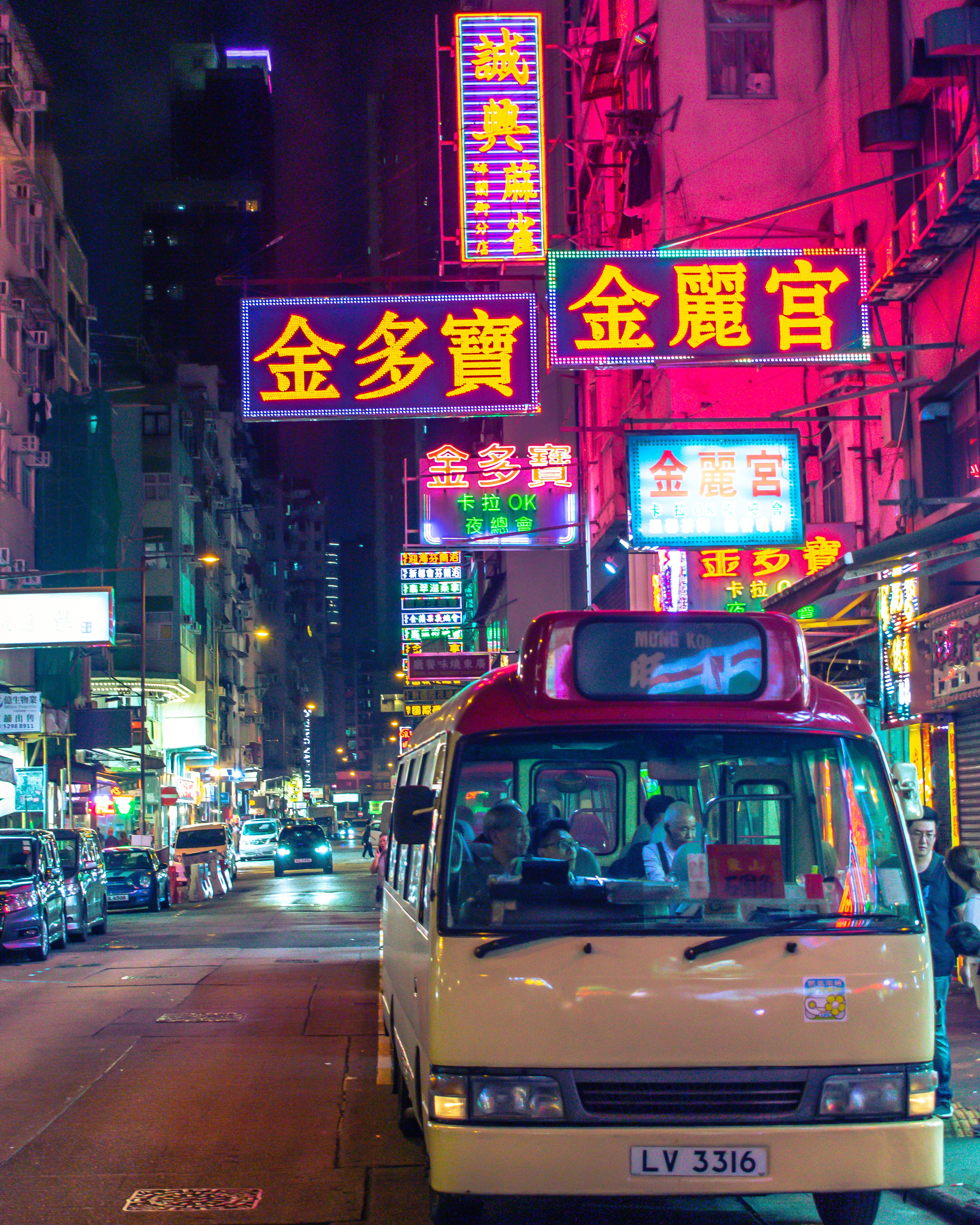
vue
<template>
<p-cropper src="./assets/sample-1.jpg" :ratio="16/9" />
</template>
Rounded Crop
Add prop rounded
to enable circular cropping.
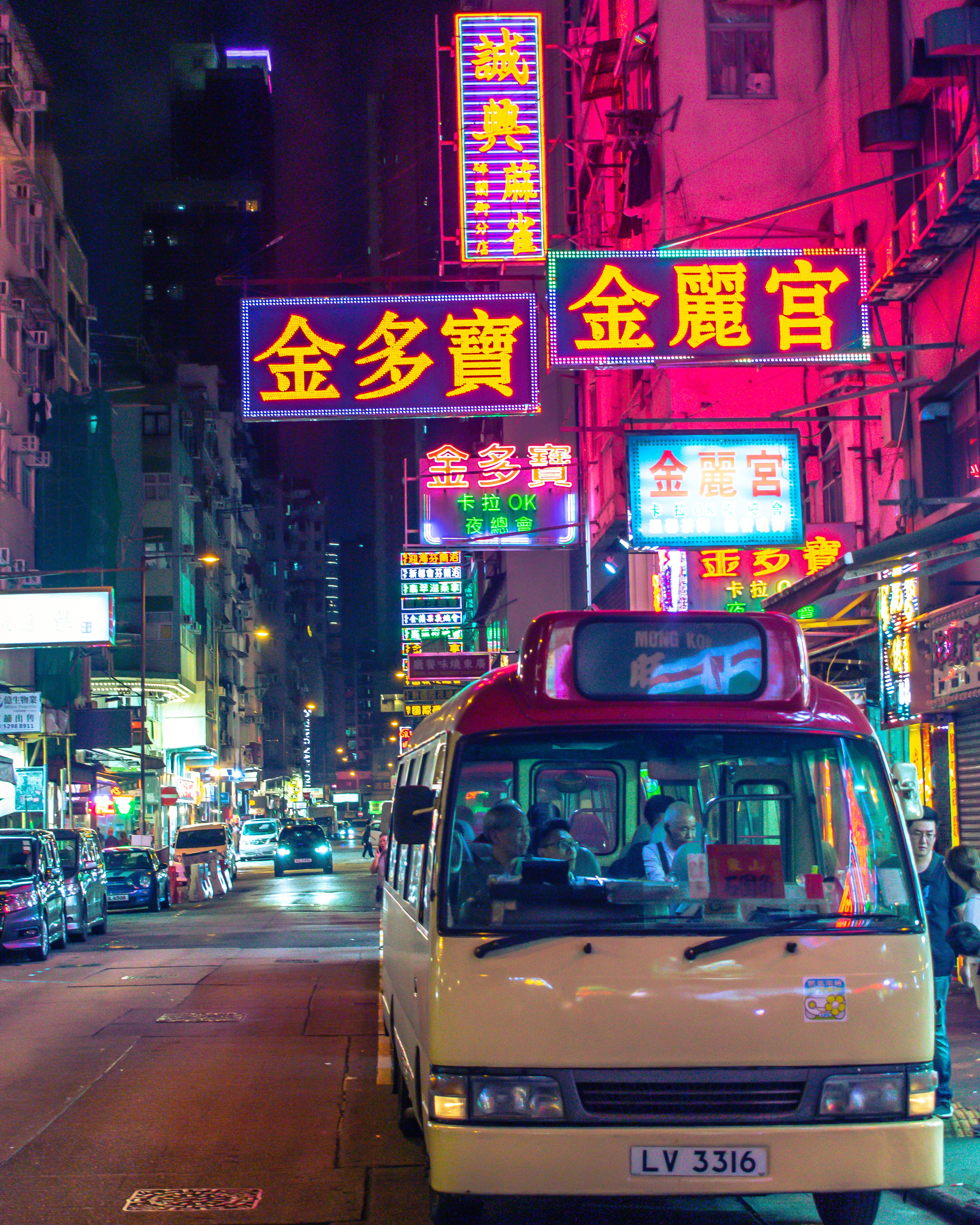
vue
<template>
<p-cropper src="./assets/sample-1.jpg" rounded />
</template>
Viewer Mode
If you want to use this component for previewing image only, you can add prop no-crop
to disabled cropping.
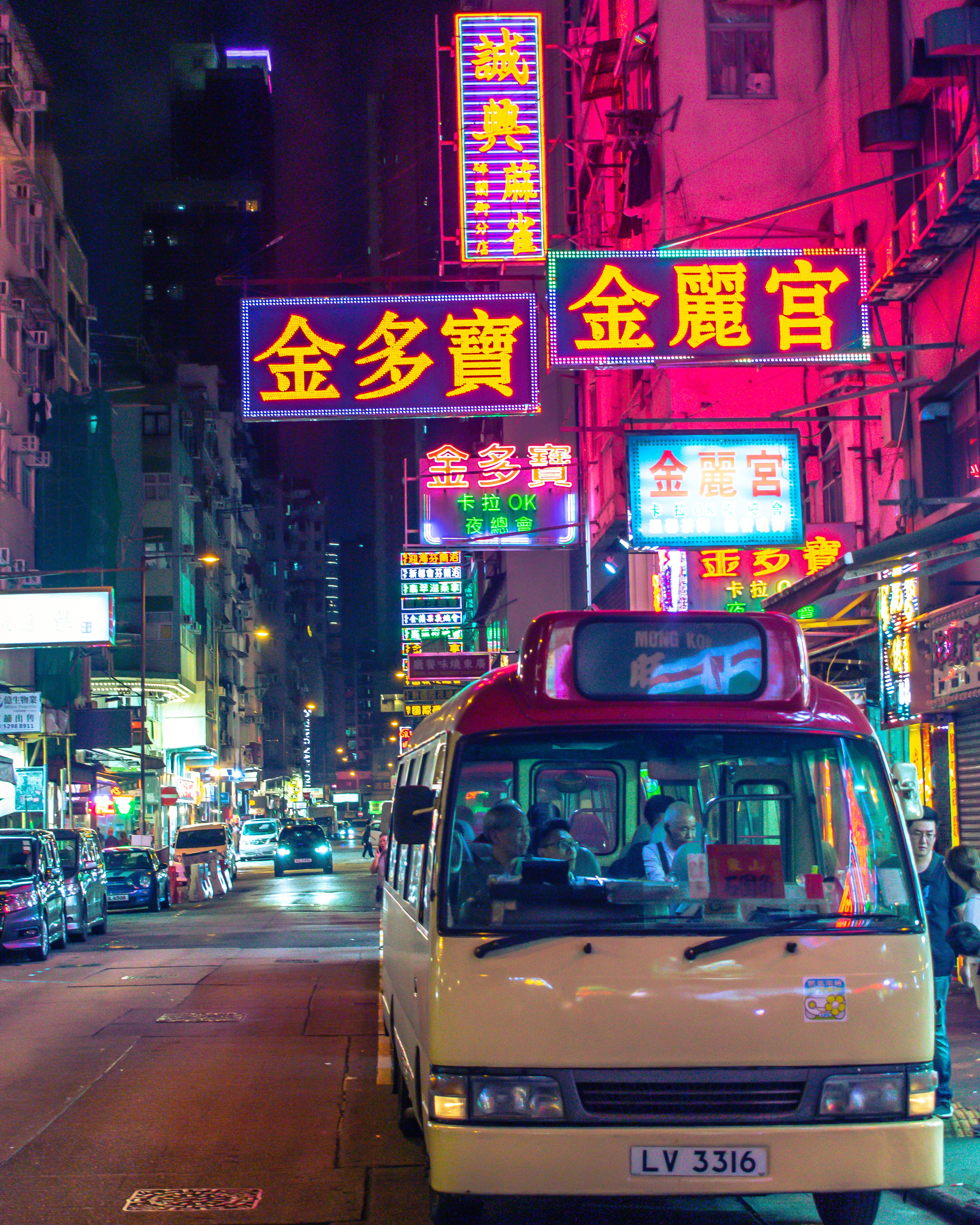
vue
<template>
<p-cropper src="./assets/sample-1.jpg" no-crop />
</template>
Binding v-model
You can bind the result of cropped image using v-model
.
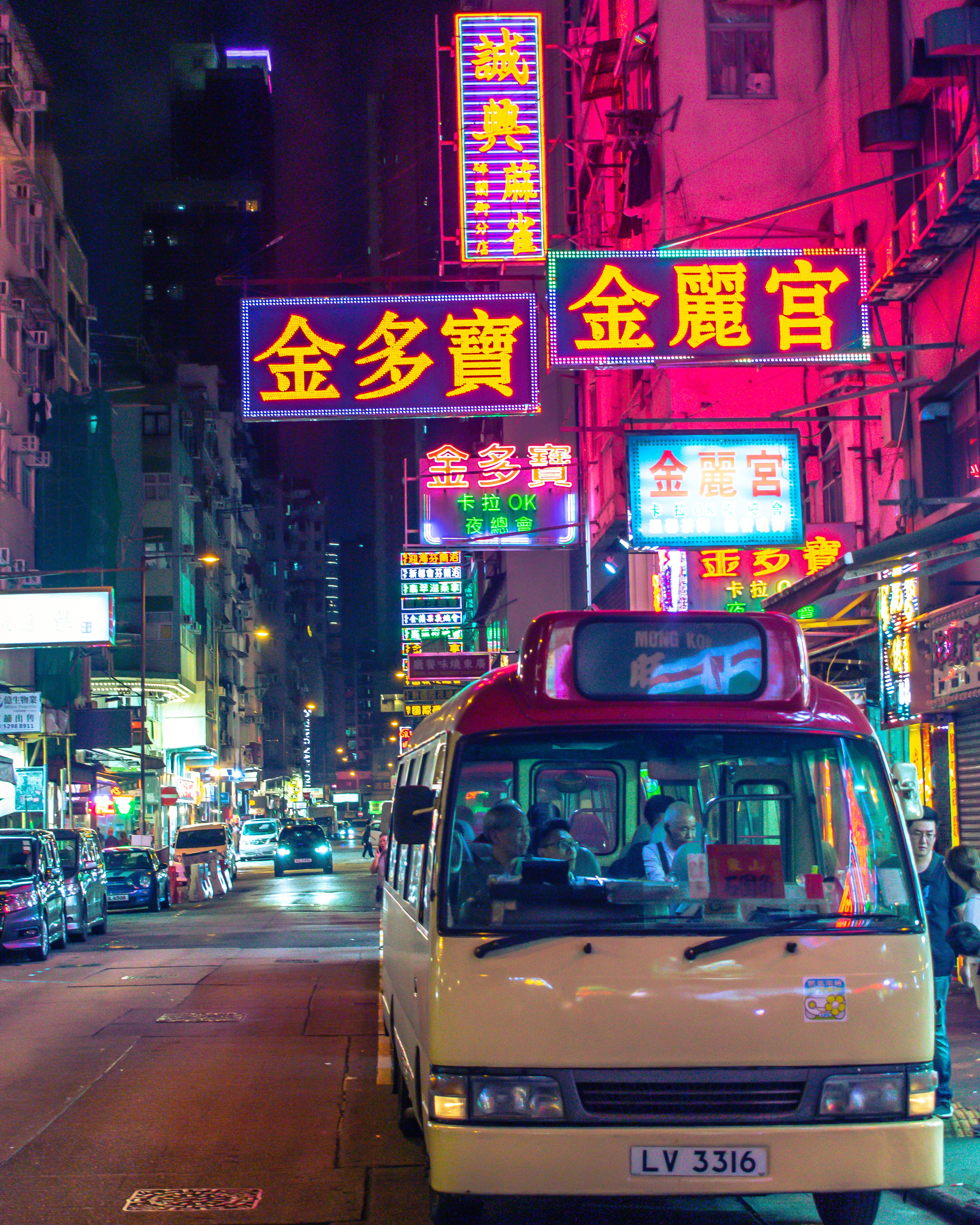
vue
<template>
<p-cropper src="./assets/sample-1.jpg" v-model="result" />
</template>
result:
Encode to base64
You can add modifier base64
to your v-model
, it's enforce result to base64-dataURI.
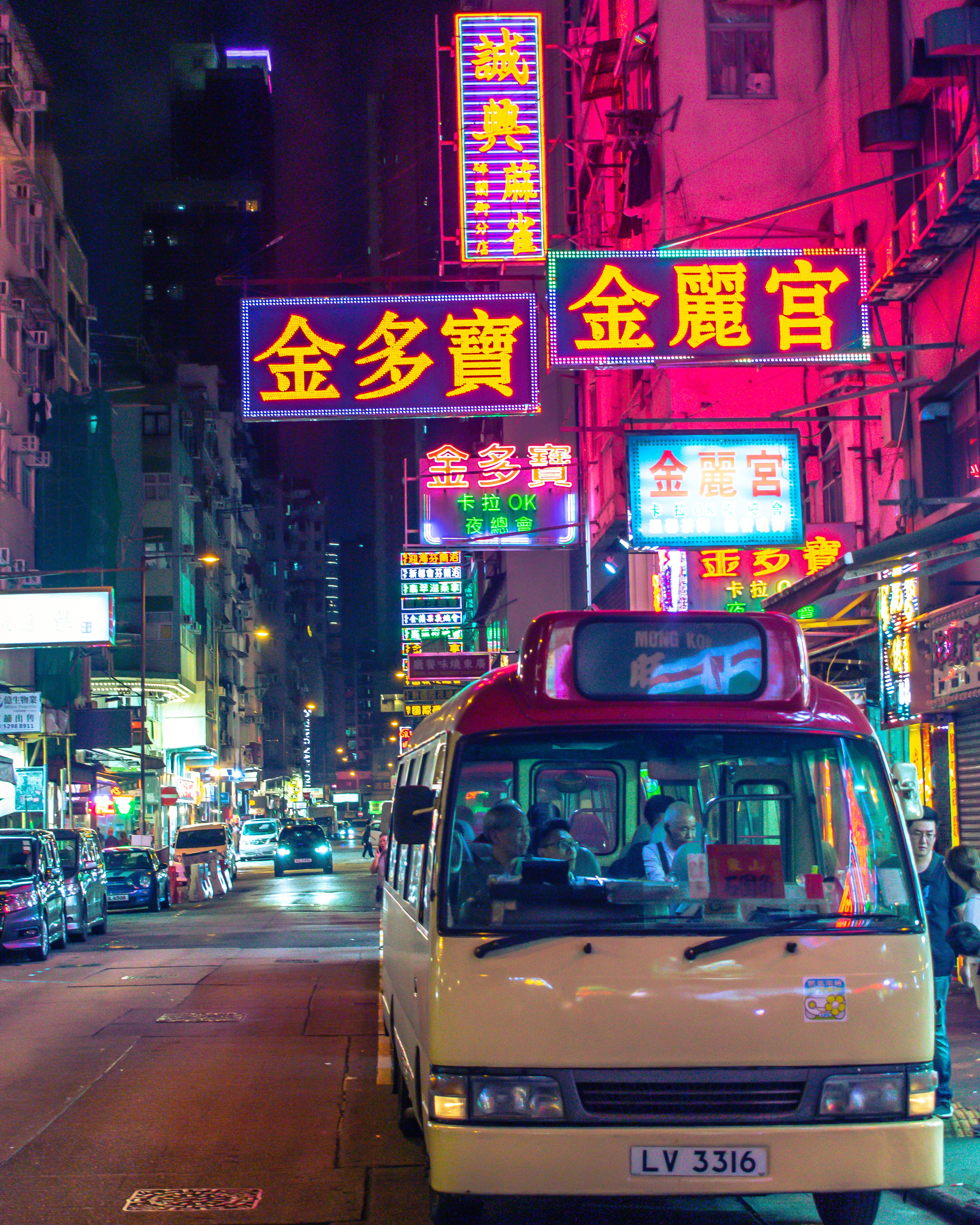
vue
<template>
<p-cropper src="./assets/sample-1.jpg" v-model.base64="result" />
</template>
result:
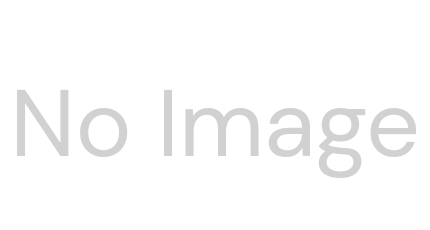
Disabling Autocrop
By default, cropping process was ran every movement (drag, zoom, & rotate). It can be exhausting the resource on some device. You can disabled it using prop no-autocrop
. And to trigger the cropping, you can use templateRef
on <p-cropper>
component, and call .crop()
function.
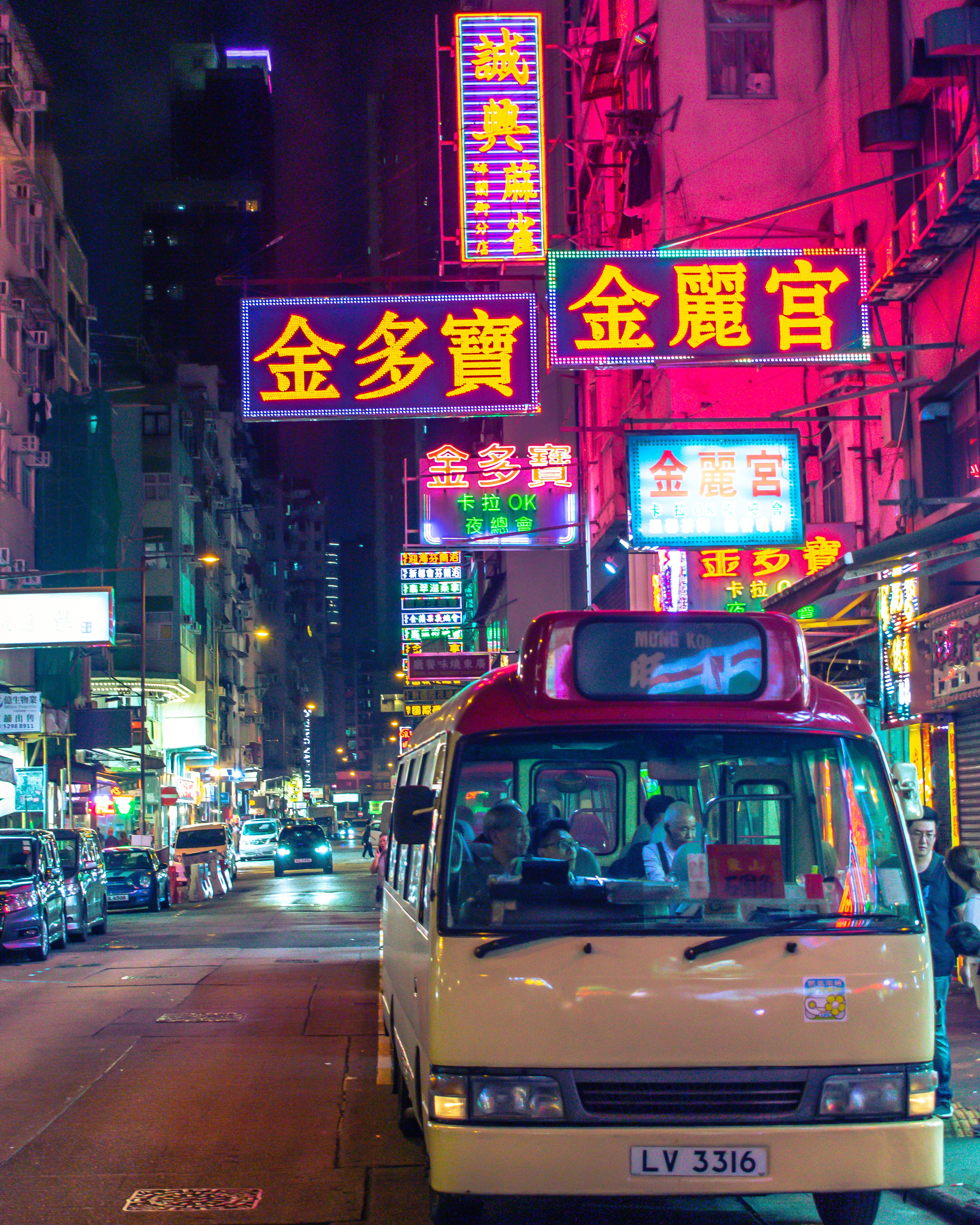
result:
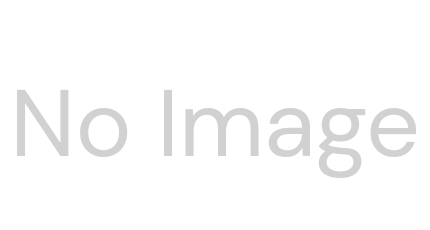
vue
<template>
<p-cropper
ref="cropper"
v-model.base64="result2"
src="./assets/sample-1.jpg"
no-autocrop />
<p-button @click="doCrop">Do Crop</p-button>
</template>
<script setup lang="ts">
const cropper = ref<InstanceType<typeof PCropper>>()
function doCrop () {
if (cropper.value)
cropper.value.crop()
}
</script>
API
Props
Props | Type | Default | Description |
---|---|---|---|
src | String , File | - | Image source |
width | Number | - | Crop width, in pixel |
height | Number | - | Crop height, in pixel |
ratio | Number | 1/1 | Crop ratio |
img-width | Number | - | Add attribute width on img tag |
img-height | Number | - | Add attribute height on img tag |
img-class | String | - | Additional class to img tag |
rounded | Boolean | false | Enabling rounded crop |
no-crop | Boolean | false | Disabling crop mode |
no-autocrop | Boolean | false | Disabling autocrop |
modelValue | File | - | v-model value binding |
Slots
Name | Description |
---|---|
control | Content for placing additional control |
Events
Name | Arguments | Description |
---|---|---|
load | - | Event when image finished loaded |
result | - | Event when cropped done |