Contextual Bar
App-level notification bar.
Usage
Simple Usage
Hey! This is Title Text and telling less
vue
<template>
<p-contextual-bar
v-model="sample"
title="Hey! This is Title Text and telling less" />
</template>
With Icon
Hey! This is Title Text and telling less
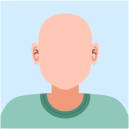
Hey! This is Title Text and telling less
vue
<template>
<p-contextual-bar v-model="sample" title="Hey! This is Title Text and telling less">
<template #icon>
<IconInfo />
</template>
</p-contextual-bar>
<p-contextual-bar v-model="sample" title="Hey! This is Title Text and telling less">
<template #icon>
<img src="../avatar/assets/avatar.png" />
</template>
</p-contextual-bar>
</template>
<script setup>
import IconInfo from '@privyid/persona-icon/vue/information-circle-solid/20.vue'
</script>
With Action Button
Hey! This is Title Text
vue
<template>
<p-contextual-bar v-model="sample" title="Hey! This is Title Text">
<template #action>
<p-button size="sm" color="info">Button text</p-button>
</template>
</p-contextual-bar>
</template>
With Two Action
This is Title Text
vue
<template>
<p-contextual-bar v-model="sample" message="This is Title Text">
<template #action>
<p-button size="sm" color="info">Button</p-button>
<p-button size="sm" color="info" variant="outline">Button</p-button>
</template>
</p-contextual-bar>
</template>
Additional Description
Hey! This is Title Text
You will be unable to sign or seal a document
vue
<template>
<p-contextual-bar
v-model="sample"
title="Hey! This is Title Text"
message="You will be unable to sign or seal a document" />
</template>
Additional Description With Action
Hey! This is Title Text
You will be unable to sign or seal a document
vue
<template>
<p-contextual-bar
v-model="sample"
title="Hey! This is Title Text"
message="You will be unable to sign or seal a document">
<template #action>
<p-button color="info" size="sm">Button</p-button>
</template>
</p-contextual-bar>
</template>
Custom Background Image

Hey! This is Title Text
You will be unable to sign or seal a document
vue
<template>
<p-contextual-bar
v-model="sample"
title="Hey! This is Title Text"
message="You will be unable to sign or seal a document"
background-url="/assets/images/img-contextualbar-bg.svg">
<template #icon>
<img src="../avatar/assets/avatar.png" />
</template>
</p-contextual-bar>
</template>
Fixed
Contextual Bar have fixed position that can be setup by using fixed
prop.
vue
<template>
<p-contextual-bar
v-model="model"
color="light"
title="Hey! This is Title Text and telling less as possible"
background-url="../../public/assets/images/img-contextualbar-bg.svg"
fixed>
<template #icon>
<img src="../avatar/assets/avatar.png" />
</template>
<template #action>
<p-button
size="sm"
color="secondary" variant="link">Cancel</p-button>
<p-button size="sm" color="info">Button Text</p-button>
</template>
<template #message>
You will be unable to sign or seal a
document while your privy balance runs out.
</template>
</p-contextual-bar>
</template>
State
Contextual Bar have 4 state variants: error
, warning
, info
and neutral
. Default is info
Hey! This is Title Text and telling less
Hey! This is Title Text and telling less
Hey! This is Title Text and telling less
Hey! This is Title Text and telling less
vue
<template>
<p-contextual-bar
state="error"
v-model="sample" title="Hey! This is Title Text and telling less" />
<p-contextual-bar
state="warning"
v-model="sample" title="Hey! This is Title Text and telling less" />
<p-contextual-bar
state="info"
v-model="sample" title="Hey! This is Title Text and telling less" />
<p-contextual-bar
state="neutral"
v-model="sample" title="Hey! This is Title Text and telling less" />
</template>
Alignment
To align Contextual Bar content, use align prop. Available value are left
, right
and center
Hey! This is Title Text
Hey! This is Title Text
Hey! This is Title Text
vue
<template>
<p-contextual-bar
align="left"
v-model="sample" title="Hey! This is Title Text" />
<p-contextual-bar
align="center"
v-model="sample" title="Hey! This is Title Text" />
<p-contextual-bar
align="right"
v-model="sample" title="Hey! This is Title Text" />
</template>

Hey! This is Title Text and telling less as possible
You will be unable to sign or seal a document while your privy balance runs out.
API
Props
Props | Type | Default | Description |
---|---|---|---|
state | String | info | Contextualbar state variants, valid value is error , warning , info and neutral |
align | String | left | Contextualbar alignment, valid value is left , center and right |
dismissable | Boolean | true | Show / Hide dismiss button |
title | String | - | Content or title inside of Contextualbar |
message | String | - | Additional message of Contextualbar |
background-url | String | - | Custom background image of Contextualbar |
background-dark-url | String | - | Custom background image of Contextualbar in Dark Mode |
fixed | Boolean | false | Activate fixed Contextualbar |
Slots
Name | Description |
---|---|
title | Title content to place in Contextualbar |
message | Additional message content to place in Contextualbar |
icon | Content to place icon in Contextualbar |
action | Content to place button in Contextualbar |
Events
Name | Arguments | Description |
---|---|---|
close | - | Event when close button clicked |
show | - | Event Contextualbar shown |
hide | - | Event Contextualbar hidden |